Why you should use Qt/QML for your next cross-platform application — part 1 — desktop
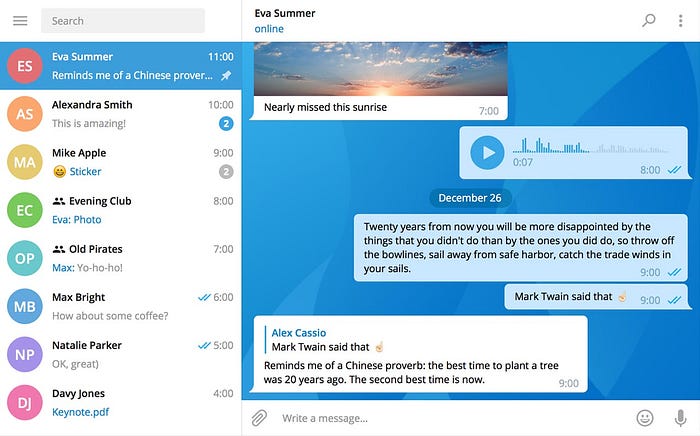
part 1 — Desktop
part 2 — Mobile
part 3 — TV
My software development story started about 16 years ago with a Pascal language developing a small console 2D game.
A lot has changed since then and today we have HTML (still) being promoted as the one technology to rule them being able to run on any platform. And while I was always somewhere on that bandwagon I’m still not happy with the current state. In the meantime, let me introduce you to another contender that I’ve been using actively for the past 5 years.
So, you (or well…your boss) is thinking about great new app that you should publish for the desktop and base your business upon. It’s yet another cool messaging app. Or maybe you were finally given the chance to rewrite that old app that brings all the money but nobody enjoys working on it since technical debt is just too big. Well, before everything, all the best!
You probably investigated or even written your app using some of the (HTML) cross platform frameworks and you are not 100% convinced about what is the right way to do it. After all, with so many options these days, which is really the way to go?
After using Qt for almost 10 years, I realized that many would like to try a completely new framework without even looking or knowing what Qt provides.
So today we are going to list some reasons on why you should try Qt/QML for your next app development.
About Qt/QML
So what is QT and what is QML exactly?
Qt is a cross-platform software development framework being used to create native embedded, desktop and mobile applications. It is written in C++which implements a wide range of different features you might need in your platform. These are developed for each platform by using native technology available on that platform.
This means that, for example, creating a audio playback app using MediaPlayer QML element will use Media Foundation on Windows while using GStreamer on Linux (see Qt Multimedia Backends).
Functionality and features are implemented and separated as modules and you can find up to date list at http://doc.qt.io/qt-5/qtmodules.html.
That gives you complete control if you want to create Websocket server to be used for your high performance application, or develop a game running on any supported platform.
There is also official Qt for python for those with a different language preference or take a look at the list of bindings to find others https://wiki.qt.io/Language_Bindings.
GUI modules
Qt supports two main ways of writing GUI applications. One is using a Widget module, and the other is using a Quick module.
Widgets provide and older way of writing GUI applications using C++ while the Quick module is a newer way using QML language and the one we will be promoting here. If you want to know more you can learn by using provided links. But keep in mind that there is no need to know or touch Widgets module if you plan to use Quick module with QML.
QML is a declarative language that is part of Qt framework and Quick module and it enables building fluid and performant user interfaces. It is declarative language specifically designed for creating graphical user interfaces which is shown every minute of it’s usage. When needed, it can be easily extended using rest of the Qt framework. Also, JavaScript support is builtin which, given the popularity of the language, is a big plus.
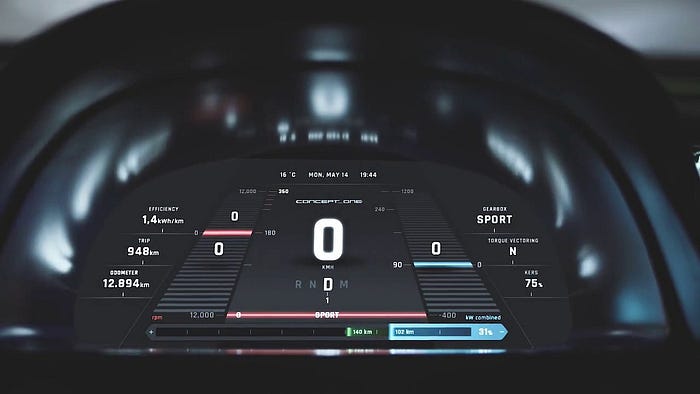
Show me the code
Now, there is nothing better to get some idea of using a framework than to see some code.
// app.qml
import QtQuick 2.10
import QtQuick.Window 2.10
import QtQuick.Controls 2.10
import QtQuick.Layouts 1.1
Window {
width: 800
height: 600
color: "black"
ColumnLayout {
anchors.fill: parent
Image {
id: image
source: "https://picsum.photos/800/600"
fillMode: Image.PreserveAspectCrop
Layout.fillHeight: true
Layout.fillWidth: true
}
Button {
text: "Quit"
Layout.alignment: Qt.AlignHCenter
onClicked: {
console.log("hello from Javascript")
Qt.quit()
}
}
}
}
So, yes, this is probably completely different to what you might have used up until now, given that it is not an existing language. (Although, personally, the latest Flutter reminds me of this)
You can use qmlscene that is part of Qt (which you can easily install in any distribution) to run this code — if you get some errors that modules are not installed, while you do have them installed, just try lowering the imported version as you are likely using older Qt version.
You can always download the latest Qt with Qt Creator from official repos and build this as a proper app - http://doc.qt.io/qt-5/qtdoc-tutorials-alarms-example.html. With this you can also get all the official examples ready to build and run.
Do you have any idea how the app will look just by reading the code?

Why you should use it
Maturity
QT framework has been around for more than 20 years while QML itself has appeared almost 10 years ago. During that time the framework has gone through quite a few iterations and was constantly improved.
Being used that much in a production also gave the needed testing from which comes stability and that you cannot get with a brand new framework which might get completely rewritten or abandoned next year.
Although some might consider that breaking APIs with each new release is cool and needed for advancements, from developer perspective, you will really appreciate the effort put in Qt that keeps it backward compatible.
On the downside, there is no official package manager/repository that would replace something you got used to, like NPM. And from my perspective, this is the only real limitation of the platform.
This means that you might be reinventing the wheel instead of getting something readily available from the internet. This will however, force you to know the code you are using which, from a performance and security perspective, might be a big plus.
Documentation & examples
You might have seen a completely new framework released yesterday. But when you are about to start using it, you realize there is not that much information on how to do anything, or it might be outdated if you rely only on examples you find on the internet.
In my experience, after using Qt for almost 10 years, it has one of the best documentations available. Every part of the framework is covered. You open the docs and start reading about creating a simple QML app and you end up in the QML scene graph explanation reading about rendering loop.
And while you might find some piece of code in the docs that doesn’t provide enough documentation for you, you will probably find complete example related to this area. Just looking at the list of examples you can see there is a lot to find for each area http://doc.qt.io/qt-5/qtexamplesandtutorials.html.
On the downside, the documentation is actually overwhelming at first. And after you get used to the level of information you get, you will have a hard time accepting documentation from other frameworks.
Javascript
Undeniably, JavaScript is a popular language so having it natively supported in QML is an obvious advantage, especially if you already have people who are working with it. This means that probably all your business logic will be written in JavaScript so you might be even able to reuse existing libraries if you have any. And if you have some heavy logic and JavaScript proves to be too slow, you can always rewrite that part using C++.
More information on using Javascript with QML can be found in the official docs.
On the downside, this might not be the 100% same JavaScript you are writing today since some parts come from your environment and are not part of the ECMAScript Language Specification. For other differences, please see http://doc.qt.io/qt-5/qtqml-javascript-hostenvironment.html.
Latest ECMAScript standards are also slower to come to QML and external libraries will probably need modifications before you can just use them as they are mostly based around Web browser and Node.js environment.
Ease of use
Now, many frameworks are popping up frequently and we have some that have been here for quite some time. But, most of them are using existing technology to do UI development. And while this does work how many of these were intended to be used for UI development?
In our experience, people are really quick to learn QML, especially those who already know JavaScript and some modern UI frameworks. They all agree, no matter the previous framework they used, that this is more natural and simplified way to write UI apps. And once they get up to speed they are much more productive as there is less boilerplate and framework doesn’t go in their way.
On the downside, there is not so many QML developers as there is HTML (pick your framework) ones so there is an expected learning curve for those who are new to the framework.
Performance
Really. Qt is after all written in C++ and it runs natively on supported platforms. And while we might have long discussions on how HTML frameworks are getting more and more performant and electron is good enough when used properly, there is just more overhead you cannot ignore.
And if you really want to go your HTML route in the future, hey, you have this covered by Qt WebEngine which integrates chromium engine in the same way while still giving you the whole Qt platform underneath that you can use to extend functionality.
Cross-platform development
Qt officially supports Windows, WinRT (Universal Windows Platform 10), MacOS, Linux, Android, iOS, tvOS and watchOS. This means that you can use it to develop your mobile and desktop apps or even an Xbox game!
If you are just starting small and have to support more than one platform or you expect to support more than one in the future, this could be the best way to do it. I worked in company where we used it to developed mobile Android/iOS apps and also apps for the AndroidTV. Comparing this to a company where we use a different technology for every single platform, there are obvious benefits.
With one technology you can share knowledge, people, programming language and even libraries.
On the downside, not every platform will support every feature you might need. Or you might already have teams with expertise on each platform, so switching frameworks could be harder.
QtCreator
Qt comes with QtCreator as it’s own IDE that you can use for Qt/QML development and is something I personally use. It has great integration with the whole Qt platform and eases development and testing on multiple platforms so they are really just a click away.
Debugger and profiler are nicely integrated so it’s really easy to quickly understand how your application behaves and where might be the bottleneck. It also provides you with a Qt Quick Designer that you can use to help your development, although, I have yet to try that.
On the downside, this is probably not the same IDE that you now use and love. If you are using some other IDE then having support for the QML will be harder (i.e. https://github.com/bbenoist/vscode-qml — which covers just the syntax) so you will need to configure the shortcuts to run CLI commands yourself.
For those who, like me, cannot switch from (neo)VIM there is also a FakeVim to compensate, although it is not really the same.
Mobile platforms?
Qt is more than ready for mobile app development for which there is a dedicated article!
Web platform?
One thing we cannot deny that there is no web apps without HTML. But gone are the days when you actually write some plain HTML with Javascript and CSS. You are probably using some abstractions like Angular with TypeScript and Sass, which also includes Node.js.
So is there something that could produce “plain” HTML from QML app?
Qt for WebAssembly is an official effort to deploy your Qt/QML apps to the web by, obviously, using webassembly. While this is now in a technology preview phase, it is in no doubt something that will happen in the future.
Update: the future is here!
There are also alternative approaches like translating QML to HTML with projects like PureQML and QMLWeb.
Where to go from here
There is really no technology that will cover all use-cases and especially preferences. That is the reason why we have some many.
For every problem there are different solutions and is up to you to weight the pros and cons.
If you already have teams using a native technology for the each platform there is probably no reason to change it. After all, software development is much more than just a framework!
But if you are looking at some fresh options, Getting Started Programming with Qt Quick should get you going. There is also a great online book you can look into as well: https://qmlbook.github.io.
Checkout part-2 to see an example cross platform Todo app!